WooCommerce Integration With Nonprofit Choice
This guide shows how to let your customers choose where you donate to on your WooCommerce site.
Allow customers to select a nonprofit
To add a Nonprofit selection field to your checkout, we’ll add a woocommerce_after_order_notes
hook to your WooCommerce site. This will insert the field under the Shipping details in your checkout.
Add the following code to your functions.php file:
/**
* Add the nonprofit field to the checkout
*/
add_action( 'woocommerce_after_order_notes', 'nonprofit_field' );
function nonprofit_field( $checkout ) {
echo '<div id="nonprofit"><h2>' . 'Choose a nonprofit' . '</h2>';
woocommerce_form_field( 'nonprofit', array(
'type' => 'select',
'class' => array('form-row-wide'),
'options' => array('Know Your Rights Camp' => 'Know Your Rights Camp', 'Watsi' => 'Watsi', 'Feeding America' => 'Feeding America'),
'label' => 'YOUR COMPANY will donate $3 to the nonprofit of your choice.',
'placeholder' => 'Select...',
), 'Know Your Rights Camp');
echo '</div>';
}
Then, save the value of the field on the customer’s Order using the woocommerce_checkout_update_order_meta
hook.
Add the following code to your functions.php file:
/**
* Update the order meta with nonprofit value
*/
add_action( 'woocommerce_checkout_update_order_meta', 'nonprofit_update_order_meta' );
function nonprofit_update_order_meta( $order_id ) {
if ( empty( $_POST['nonprofit'] ) ) {
return;
}
// Get these Nonprofit ids from https://api.getchange.io/nonprofits.
$nonprofit_ids = array(
'Know Your Rights Camp' => 'n_XI19548HjWhTUzRUYwAQoBqt',
'Watsi' => 'n_IfEoPCaPqVsFAUI5xl0CBUOx',
'Feeding America' => 'n_ykwxyhbBfPOSnGxh6EdAq2iK',
);
$nonprofit = sanitize_text_field( $_POST['nonprofit'] );
$nonprofit_id = $nonprofit_ids[$nonprofit];
if ( empty( $nonprofit_id ) ) {
// The nonprofit is unknown. Use 'Know Your Rights Camp' as a default.
$nonprofit_id = 'n_XI19548HjWhTUzRUYwAQoBqt';
}
$order = wc_get_order( $order_id );
$order->update_meta_data( 'nonprofit_id', $nonprofit_id );
$order->save();
}
Make donation to the selected nonprofit
To make a donation for each shipment, we’ll add a woocommerce_payment_complete
hook to your WooCommerce site. This will run the specified function whenever a payment is submitted.
Add the following code to your functions.php file:
Fill in your details
Make sure to fill in the TODO
details in this code snippet.
/**
* Make a donation once payment is completed
*/
add_action( 'woocommerce_payment_complete', 'make_donation' );
function make_donation( $order_id ) {
// TODO: Configure these options.
// `amount` The amount of the donation in cents.
// `public_key` Get this from https://api.getchange.io. Test key starts with pk_test, Prod key starts with pk_live.
// `secret_key` Get this from https://api.getchange.io. Test key starts with sk_test, Prod key starts with sk_live.
$amount = 500;
$public_key = '';
$secret_key = '';
// Get the nonprofit
$order = wc_get_order( $order_id );
$nonprofit_id = $order->get_meta( 'nonprofit_id' );
// Prepare the donation
$url = 'https://api.getchange.io/api/v1/donations';
$data = array('amount' => $amount, 'nonprofit_id' => $nonprofit_id, 'funds_collected' => false);
$options = array(
'http' => array(
'header' => array("Content-type: application/json", "Authorization: Basic " . base64_encode($public_key . ":" . $secret_key)),
'method' => 'POST',
'content' => json_encode($data)
)
);
// Send the donation
$context = stream_context_create($options);
$result = file_get_contents($url, false, $context);
// Save the donation_id on the Order
$donation_id = json_decode($result, true)['id'];
$order->update_meta_data( 'donation_id', $donation_id );
$order->save();
}
Allow customers to share their donation on social media
Change generates ready-to-share social media content for each donation. Include it on your Thank You page via the woocommerce_thankyou
hook:
Fill in your details
Make sure to fill in your public_key
in this code snippet.
/**
* Add social media content sharing to the Thank You page.
*/
add_action( 'woocommerce_thankyou', 'donation_social_media_content' );
function donation_social_media_content( $order_id ) {
// TODO: Insert your public key.
$public_key = '';
$order = wc_get_order( $order_id );
$donation_id = $order->get_meta( 'donation_id' );
$nonprofit_id = $order->get_meta( 'nonprofit_id' );
if ( empty( $donation_id ) || empty( $nonprofit_id ) ) {
return;
}
// Add the social media content drop-in element to the page.
echo '<script type="module" src="https://unpkg.com/@getchange/change-social-content@0.2.0/change-social-content.min.js"></script>';
echo "<change-social-content nonprofitId='$nonprofit_id' donationId='$donation_id' publicKey='$public_key' style='max-width: 400px'></change-social-content>";
}
Customers will now have the option to share their donation on the Thank You page:
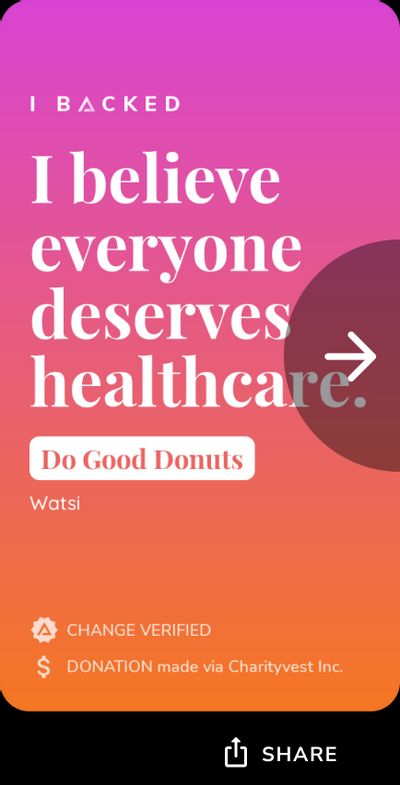
Conclusion
That’s it. When your customer completes an order, a donation will be made to the nonprofit of their choice. They’ll be presented with the option to share their donation to social media on the Order Thank You page.
To update the customers’ nonprofit choices, make sure to update the arrays in both nonprofit_field
and nonprofit_update_order_meta
.